When loading images, best practice is to show a placeholder instead of a broken image. A great place to see this in action is on Pinterest. They achieve this by showing the dominant color of the image as the background placeholder, only showing the image when it has been loaded.
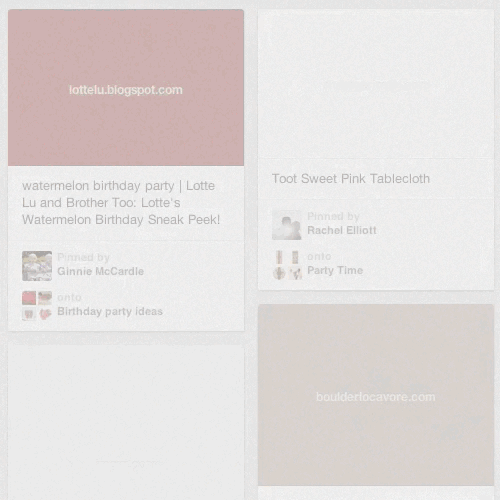
Our goal is to recreate this effect using Embedly's API. Below is a demo utilizing Extract and Display to recreate this image loading technique.
function rgbToHex(r, g, b) {
return "#" + ((1 << 24) + (r << 16) + (g << 8) + b).toString(16).slice(1);
}
function displayImage(img, color, provider) {
var width = 400,
height = Math.ceil(img.height * (width / img.width));
// Add the block to the results section.
$('.results').html(
'<div class="background-wrapper"><div id="backgroundBlock"><span>' +
provider + '</span></div></div>');
// Create a wrapper.
$('.background-wrapper').css({
width: width + 20,
height: height + 20
});
// Build the actually placeholder.
$('#backgroundBlock').css({
background: color,
width: width,
height: height
});
// Center the text.
$('#backgroundBlock span').css('padding-top', Math.floor(height / 2) - 10);
// Animate the fade in.
$('.background-wrapper').animate({
opacity: 1
}, 500);
// Use Embedly display to resize the image, we add a timestamp in the demo o
var src = $.embedly.display.resize(img.url, {
query: {
width: width,
height: height
},
key: $.embedly.defaults.key
}) +
'&_' + Math.floor(Math.random() * 1000);
// Create the img element
var elem = document.createElement('img');
// Set up the onload handler.
var loaded = false;
var handler = function() {
if (loaded) {
return;
}
loaded = true;
// Fade the image into view.
$('#backgroundBlock img').animate({
opacity: 1
}, 1000);
};
// Add the attributes to the image.
elem.onload = handler;
elem.src = src;
elem.style.display = 'block';
// Place the image in the correct ID.
document.getElementById('backgroundBlock').appendChild(elem);
// If the image is already in the browsers cache call the handler.
if (elem.complete) {
handler();
}
};
$('.collapse .button').on('click', function() {
// Grab the input.
var url = $('input').val();
// Make the call to Embedly Extract
$.embedly.extract(url)
.progress(function(obj) {
// Grab images and create the colors.
var img = obj.images[0],
hex = rgbToHex(img.colors[0].color[0], img.colors[0].color[1], img.colors[0].color[2]);
// Display the image inline.
displayImage(img, hex, obj.provider_display);
});
});
.background-wrapper {
margin: 0 auto;
}
#backgroundBlock {
position: relative;
}
#backgroundBlock span {
color: #fff;
font-weight: bold;
margin: 0 auto;
text-align: center;
display: block;
}
#backgroundBlock img {
position: absolute;
top: 0;
left: 0;
opacity: 0;
}